Embedding Superset dashboards in React applications
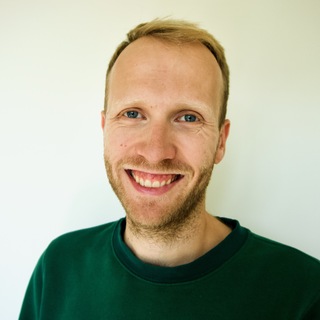
- Name
- Dennis Paagman
- @djfpaagman
Superset is an open-source data visualization platform, while Preset is a hosted SaaS solution for Superset. A notable capability of Superset is its ability to embed dashboards within other applications. This feature allows for easy dashboard management using Superset, without requiring users to leave your application. Additionally, Superset offers security rules to ensure data is correctly filtered for each user or team.
To embed dashboards, Superset provides an SDK that renders an iframe within your application. This process requires a special guest token, which you can generate on your application’s backend by interacting with the Superset API.
This article presumes that you’ve already set up and configured Superset and written the backend code to generate guest tokens. If not, please refer to the official Preset (or Superset) documentation.
The primary challenge I encountered while integrating Superset into our React application was making sure I called the embed code at the right moment and only once. The library and needs access to a DOM element to insert the iframe. However, it wouldn’t render correctly when other parts of the page were still loading (and the mounting point wouldn’t exist yet) and would suffer from unnecessary additional calls caused by re-renders.
My initial attempts used useEffect
and useLayoutEffect
hooks, which didn’t solve the problems consistently. My next attempt involved useRef
with a reference to the mounting point. However, useRef
does not trigger re-renders by design, so there’s no way to re-trigger the effect once the current reference is set after the element is added to the DOM.
Luckily, there’s an alternative process where we can pass a callback function directly to ref
. This method, known as a “ref callback function”, passes the DOM node you set the ref
on as an argument:
When the
<div>
DOM node is added to the screen, React will call yourref
callback with the DOMnode
as the argument.
<div ref={(node) => console.log(node)} />
This perfectly suits our needs as it will only be called when the element is available in the DOM.
The final point to address is to make sure that the embedding code is called only once, not every time the component is re-rendered, for example when a parent gets re-rendered. This can be achieved by keeping a simple ‘mounted’ state, which can then be used to make sure the code gets called only once.
Combining these elements, we can create a custom hook. This custom hook takes one argument, the id
of the dashboard, and returns a callback function that takes the mounting point as the sole argument. This function can then be passed into the ref
as the direct callback function in your component:
import { useCallback } from "react";
import { embedDashboard } from "@superset-ui/embedded-sdk";
// Implement your actual API backend call here (through a Promise).
const fetchGuestToken = () => { /* ... */ };
export const useSupersetEmbed = (id: string) => {
const [mounted, setMounted] = useState(false);
return async (mountPoint: HTMLDivElement | null) => {
if (!mountPoint || mounted) return;
await embedDashboard({
id,
supersetDomain: "https://....",
mountPoint,
fetchGuestToken,
// dashboardUiConfig: {},
// debug: true,
});
setMounted(true);
};
};
export const SupersetExampleDashboard = () => {
const embed = useSupersetEmbed("your-dashboard-id-here");
return <div ref={embed} />;
};
And that’s it! You can now embed Superset dashboards in your React application without any reloading or rendering issues.